You've already forked comprehensive-rust
mirror of
https://github.com/google/comprehensive-rust.git
synced 2025-06-22 00:37:34 +02:00
Added break-continue section. (#1793)
This is a contribution of a break-continue section for Comprehensive Rust. 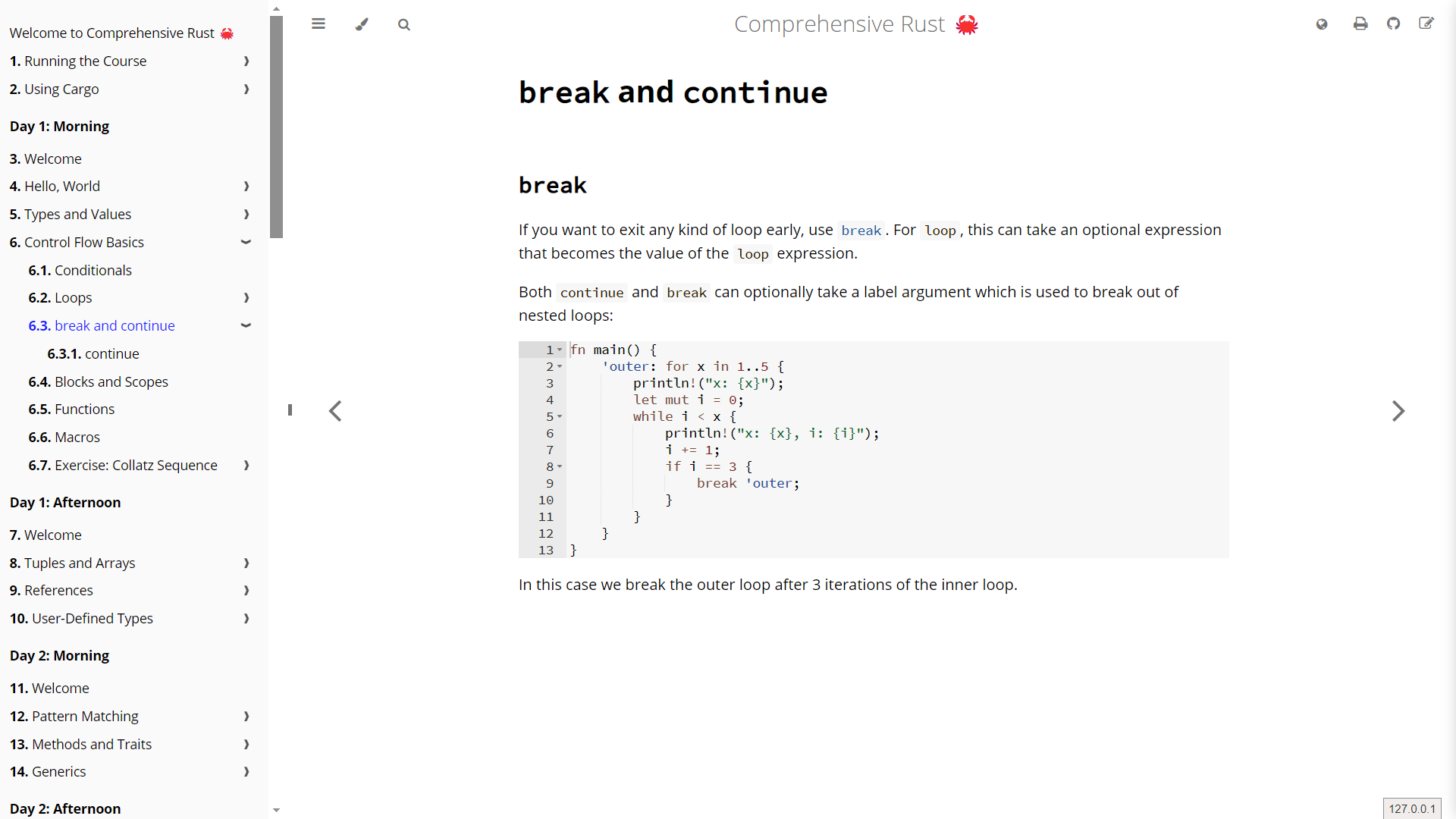  --------- Co-authored-by: Dustin J. Mitchell <dustin@v.igoro.us>
This commit is contained in:
committed by
GitHub
parent
aaef818b23
commit
f391e863ab
@ -35,6 +35,7 @@
|
||||
- [`for`](control-flow-basics/loops/for.md)
|
||||
- [`loop`](control-flow-basics/loops/loop.md)
|
||||
- [`break` and `continue`](control-flow-basics/break-continue.md)
|
||||
- [Labels](control-flow-basics/break-continue/labels.md)
|
||||
- [Blocks and Scopes](control-flow-basics/blocks-and-scopes.md)
|
||||
- [Functions](control-flow-basics/functions.md)
|
||||
- [Macros](control-flow-basics/macros.md)
|
||||
|
@ -4,56 +4,26 @@ minutes: 4
|
||||
|
||||
# `break` and `continue`
|
||||
|
||||
If you want to immediately start the next iteration use
|
||||
[`continue`](https://doc.rust-lang.org/reference/expressions/loop-expr.html#continue-expressions).
|
||||
|
||||
If you want to exit any kind of loop early, use
|
||||
[`break`](https://doc.rust-lang.org/reference/expressions/loop-expr.html#break-expressions).
|
||||
For `loop`, this can take an optional expression that becomes the value of the
|
||||
`loop` expression.
|
||||
|
||||
If you want to immediately start the next iteration use
|
||||
[`continue`](https://doc.rust-lang.org/reference/expressions/loop-expr.html#continue-expressions).
|
||||
|
||||
```rust,editable
|
||||
fn main() {
|
||||
let (mut a, mut b) = (100, 52);
|
||||
let result = loop {
|
||||
if a == b {
|
||||
break a;
|
||||
let mut i = 0;
|
||||
loop {
|
||||
i += 1;
|
||||
if i > 5 {
|
||||
break;
|
||||
}
|
||||
if a < b {
|
||||
b -= a;
|
||||
} else {
|
||||
a -= b;
|
||||
}
|
||||
};
|
||||
println!("{result}");
|
||||
}
|
||||
```
|
||||
|
||||
Both `continue` and `break` can optionally take a label argument which is used
|
||||
to break out of nested loops:
|
||||
|
||||
```rust,editable
|
||||
fn main() {
|
||||
'outer: for x in 1..5 {
|
||||
println!("x: {x}");
|
||||
let mut i = 0;
|
||||
while i < x {
|
||||
println!("x: {x}, i: {i}");
|
||||
i += 1;
|
||||
if i == 3 {
|
||||
break 'outer;
|
||||
}
|
||||
if i % 2 == 0 {
|
||||
continue;
|
||||
}
|
||||
println!("{}", i);
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
In this case we break the outer loop after 3 iterations of the inner loop.
|
||||
|
||||
<details>
|
||||
|
||||
- Note that `loop` is the only looping construct which returns a non-trivial
|
||||
value. This is because it's guaranteed to be entered at least once (unlike
|
||||
`while` and `for` loops).
|
||||
|
||||
</details>
|
||||
|
29
src/control-flow-basics/break-continue/labels.md
Normal file
29
src/control-flow-basics/break-continue/labels.md
Normal file
@ -0,0 +1,29 @@
|
||||
# Labels
|
||||
|
||||
Both `continue` and `break` can optionally take a label argument which is used
|
||||
to break out of nested loops:
|
||||
|
||||
```rust,editable
|
||||
fn main() {
|
||||
let s = [[5, 6, 7], [8, 9, 10], [21, 15, 32]];
|
||||
let mut found = false;
|
||||
let target_value = 10;
|
||||
'outer: for i in 0..=2 {
|
||||
for j in 0..=2 {
|
||||
if s[i][j] == target_value {
|
||||
found = true;
|
||||
break 'outer;
|
||||
}
|
||||
}
|
||||
}
|
||||
print!("{}", found)
|
||||
}
|
||||
```
|
||||
|
||||
<details>
|
||||
|
||||
- Note that `loop` is the only looping construct which returns a non-trivial
|
||||
value. This is because it's guaranteed to be entered at least once (unlike
|
||||
`while` and `for` loops).
|
||||
|
||||
</details>
|
Reference in New Issue
Block a user